I’m going to start a series of tutorials over the next weeks and months about HTML5. A lot of web developers are not leveraging HTML5 for a variety of reasons. We have been so trained over the past decade to embrace XHTML 1.0 that we’ve avoided the new DOCTYPE as something new that needs to be learned.
The good news is, XHTML is still valid in HTML5. The better news is now you have much more fun toys to play with.
Admittedly, I was one of those people who delayed jumping on the HTML5 bandwagon. In the past few months, however, that has changed. This series of articles will hopefully help the web developer to rethink how they develop on the web. Much of the stuff I’m about to talk about does not require a lot of extra heavy lifting.
Use the Correct DOCTYPE
Just as a remedial exercise of laying out the premise, your HTML must have the correct DOCTYPE. In XHTML 1.0/1.1, the first line of the HTML page had to be something along these lines
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
That’s relatively confusing, huh? Makes you want to go drink spoiled milk with lumpy crud in it just because it’s tasty, right?
To declare a web page as HTML5, you do the same thing you did with the old 1990s era HTML4, before the web embarked on the XHTML idea of doing work. HTML5 is, essentially, a reset to HTML4 with all kinds of additional goodness. You simply start a web document with:
<!DOCTYPE html>
A lot easier, right? Heck, you can type that in your sleep once you’ve typed it enough (I know you already do that with your drivers license and credit card numbers).
Form Field Types
With all that remedial knowledge in play, let’s take a look at HTML5 forms. The importance of this might be lost if the only thing you think about, when building HTML pages, are computer browsers. But if you recognize we live in a mobile world, you own an Android or iPhone and have tried to do any kind of form filling on those devices, then you might start to realize the importance of field types.
In XHTML, you might have a form that looks like this:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | <form action="" method="post"> <label for="name">Full Name: <input type="text" name="name" id="name" /> </label> <label for="phone">Phone Number: <input type="text" name="phone" id="phone" /> </label> <label for="email">Email Address: <input type="text" name="email" id="email" /> </label> <label for="website">Web URL: <input type="text" name="website" id="website" /> </label> </form> |
In XHTML, we didn’t have a lot of field types. We had text (which everything above is), hidden, password (*’s entered in the input), checkboxes and radio buttons. You could add other types of inputs (That don’t use the <input>
tag and include <select>
and <textarea>
.
This works in HTML5 too, but you’re limited by one default keyboard – which is fine, but fairly bland and not at all contextual.
What would happen if we changed that form to use different field types? HTML5 support a bunch. The four fields above can more sematically have the following types, in order: text, tel, email, url.
Watch what happens on the iPhone when the HTML looks like this (Android is similar):
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | <form action="" method="post"> <label for="name">Full Name: <input type="text" name="name" id="name" /> </label> <label for="phone">Phone Number: <input type="tel" name="phone" id="phone" /> </label> <label for="email">Email Address: <input type="email" name="email" id="email" /> </label> <label for="website">Web URL: <input type="url" name="website" id="website" /> </label> </form> |
For a standard text field, your keyboard will look like this:
For a phone number, using the tel
type:
For an email address, using the email
type:
And for a URL field using the url
type:
There are, of course, other field types that I’m not going to go into too much here. But to whet your appetite, there is a color
type that attaches to a color picker. There’s a date
type that binds to a date picker. There’s even a range
type which binds to a slider picker.
Important: Not all browsers support all types. In the event that a browser does not support a specific type, it falls back to standard text
type and you can bind other external Javascript to make the same functionality happen.
Reference: All HTML5 field types with browser support.
Form Field Placeholder
Another useful feature is the placeholder. In XHTML, we might have a form that looks like this:
1 2 3 |
This would create a simple field that would be pre-populated with “Search for your term”. From a usability standpoint, when a user brings that field into focus, the text is supposed to disappear and allow the typing of a search term. If nothing is typed and the focus is switched to a different element, then that phrase should re-appear.
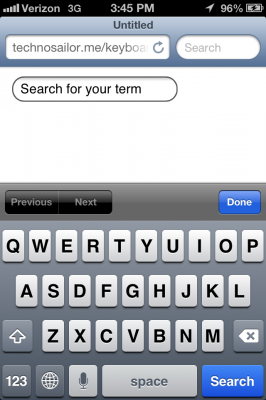
You can’t do this without a little Javascript help (I like jQuery for this) – which is outside the scope of this article. But in HTML5, you only have to add placeholder="Search for your term"
. Different browsers handle this slightly different, but they all insert some dummy text that can be replaced by the user’s own input.
1 2 3 |
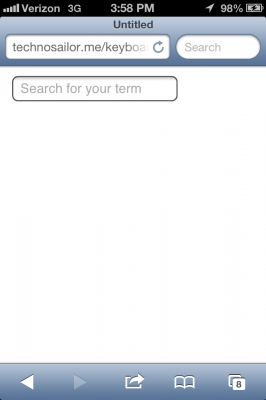
Form Field Validation
Validation is such a tedious thing for developers. You can do all kinds of ugly things to make sure fields that are required actually have a value or that a field meets a certain criteria (for instance, a zip code field having 5 numeric characters to match the U.S. format).
In terms of requiring a field, it’s as simple as adding required
to the input tag. In HTML5, you don’t have to have an explicit value for an attribute as you do in XHTML. You can if you want, type <input type="text" name="zip" required="required" />
but this is a habit that does not need muscle exercise. Just use:
1 2 3 4 |
Let’s validate that zip code field, though, because this is where HTML5 really shines.
Using the pattern
attribute, you can designate a regular expression to match formatting needs. If we want to limit the zip field to 5 numbers (most simplistic example – it could also have a potential extra dash and 4 numbers too), you might use this HTML:
1 2 3 4 |
You can find a list of helpful regexes at html5pattern.com.
Summary
There’s a lot more we could get into here, but the point of this exercise is to prove that it doesn’t take much to start using HTML5 in development. Doing so will also push the boundaries of what has been more commonly possible and the barrier to entry is so low that I struggle to find a reason why HTML5 should not be used more commonly.
I’ll have more of these in the days and weeks to come so stay tuned, subscribe to the RSS feed and, as always, if you’re interested in hiring me for a full time gig or contract basis, please reach out to me. I am actively looking.